Graphs
A graph is an object made of two (TGraph
) or three
(TGraph2D
) arrays X
, Y
and Z
holding the x
,y
and z
coordinates of n points.
Un-binned data
A graph or chart is a set of categorical variables, this is un-binned data unlike a histogram which holds continuous data, where the bins represent ranges of data (binned data), see → Histograms.
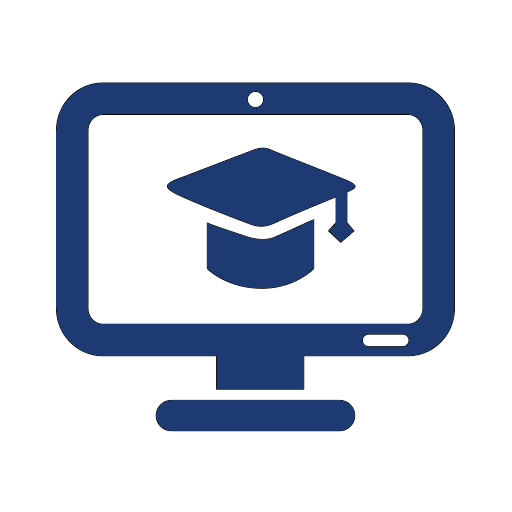
Graph classes
ROOT provides numerous graph classes:
X-Y graphs
TGraph: A graph.
TGraphErrors: A graph with symmetric error bars.
TGraphAsymmErrors: A graph with asymmetric error bars.
TGraphBentErrors: A TGraph
with bent, asymmetric error bars.
TGraphMultiErrors: A TGraph
with asymmetric error bars and multiple y error dimensions.
TGraphPolar: A TGraphErrors
represented in polar coordinates.
TGraphQQ: Draws quantile-quantile plots.
TMultiGraph: A collection of TGraph
(or derived) objects.
X-Y-Z graphs
TGraph2D: A graph made of three arrays X, Y and Z with the same number of points each.
TGraph2DErrors: A TGraph2D
with error bars.
Working with TGraph
This section explains how to work with a TGraph
an its
derived classes. It covers the creation,
the drawing and the
fitting.
Creating a graph
- Use one of the TGraph constructor to create a graph object.
TGraph
, and its derived classes, offers a wide variety of constructors.
A TGraph
can be created from an ASCII file, from a TF1
, from a histogram etc …
The most common way being the creation from C++ arrays.
Example
Arrays of coordinates are defined and then the graph is created with
the TGraph
constructor.
The coordinates can be arrays of doubles or floats.
int n = 20;
double x[n], y[n];
for (int i=0; i<n; i++) {
x[i] = i*0.1;
y[i] = 10*sin(x[i]+0.2);
}
auto gr = new TGraph (n, x, y);
When the number of points to be stored in the graph is not known AddPoint can be used:
auto gr = new TGraph();
for (int i=0; i<20; i++) gr->AddPoint(i*0.1, 10*sin(i*0.1+0.2));
Drawing a graph
- Use the TGraph::Draw() method to draw a graph. Several drawing options are available.
Example
auto gr = new TGraph();
for (int i=0; i<20; i++) gr->AddPoint(i*0.1, 10*sin(i*0.1+0.2));
gr->Draw();
Figure: Graph drawn with Draw().
The “drawing option” is the unique parameter (case insensitive) of the TGraph::Draw() method. It specifies how the graph will be graphically rendered.
Example
auto gr = new TGraph();
for (int i=0; i<20; i++) gr->AddPoint(i*0.1, 10*sin(i*0.1+0.2));
gr->Draw("AL*"); // Draw() specifies the drawing option.
Figure: Graph drawn with Draw(“AL*”).
Setting titles for a graph
On the previous plot the graph title is “Graph”. It is the default graph title. The axis have no titles. Once a graph is created it is possible to change the graph and axis titles using TGraph::SetTitle() method.
You can use the TAxis::CenterTitle method to center the title. Note this acts on the axis which are available only once the graph has been drawn.
Example
{
auto gr = new TGraph();
gr->SetTitle("Graph title;X-Axis;Y-Axis");
for (int i=0; i<20; i++) gr->AddPoint(i*0.1, 10*sin(i*0.1+0.2));
gr->Draw();
gr->GetXaxis()->CenterTitle()
}
Figure: Graph with titles.
Zooming a graph
To zoom a graph you can first draw a frame with the wanted limits and then draw the graph in it
Example
{
auto c = new TCanvas("c","A Zoomed Graph",200,10,700,500);
c->DrawFrame(0,1,0.5,8);
int n = 10;
double x[10] = {-.22,.05,.25,.35,.5,.61,.7,.85,.89,.95};
double y[10] = {1,2.9,5.6,7.4,9,9.6,8.7,6.3,4.5,1};
auto gr = new TGraph(n,x,y);
gr->SetMarkerColor(4);
gr->SetMarkerStyle(20);
gr->Draw("LP");
}
Figure: A zoomed graph.
See also “How to set ranges on axis”
Fitting graphs
- Use the graph
Fit()
methods (for example TGraph::Fit()), for fitting graphs.
For more information on the Fit()
method, → see Fitting histograms.
Graphs with error bars
A TGraphErrors
is a TGraph
with error bars.
Several drawing options are available for graphs with error bars.
TGraphErrors
uses 4 parameters: X, Y (as in TGraph
), X-errors, and Y-errors (the size of the errors in the x and y direction).
When the errors are not symmetric use TGraphAsymmErrors
. It
uses 4 vectors to define the errors. The drawing options are the same as those for TGraphErrors
TGraphBentErrors
is similar to TGraphAsymmErrors
but it has
4 extra parameters to shift (bent) the error bars. This is useful when several graphs
are drawn on the same plot and may hide each other.
TGraphMultiErrors
is an other kind of % include ref class=”TGraphAsymmErrors” %} but
multiple y error dimensions. It has a set of specific drawing options.
TGraphPolar
is an other kind ot graph with errors. It allows
to produce polar plots with several plotting options.
Graphs with exclusion zone
It is possible to draw a graph with an exclusion zone.
When drawn with options C
or L
one side of the graph is hatched.
Reverse axis
Sometimes it is useful to draw the axis “reversed”. Ie: the X axis with its minimum on the right and its maximum on left of the plot and the Y axis its minimum on top and its maximum at the bottom of the plot. Some special drawing options allow to do that.
Logarithmic scale
Logarithmic scales can be selected for the X and Y axis of a graph. But only the points building the graphs are changed into log scale. Not the lines connecting them.
TMultiGraph
A TMultiGraph
is a collection of TGraph
(or derived) objects.
Creating a TMultiGraph
- Use TMultiGraph::Add() to add a new graph to the list.
The TMultiGraph
owns the objects in the list. The drawing and
fitting options are the same as for TGraph.
Example
{
// Create the points:
const int n = 10;
double x[n] = {-.22,.05,.25,.35,.5,.61,.7,.85,.89,.95};
double y[n] = {1,2.9,5.6,7.4,9,9.6,8.7,6.3,4.5,1};
double x2[n] = {-.12,.15,.35,.45,.6,.71,.8,.95,.99,1.05};
double y2[n] = {1,2.9,5.6,7.4,9,9.6,8.7,6.3,4.5,1};
// Create the width of errors in x and y direction:
double ex[n] = {.05,.1,.07,.07,.04,.05,.06,.07,.08,.05};
double ey[n] = {.8,.7,.6,.5,.4,.4,.5,.6,.7,.8};
// Create two graphs:
TGraph *gr1 = new TGraph(n,x2,y2);
TGraphErrors *gr2 = new TGraphErrors(n,x,y,ex,ey);
// Create a TMultiGraph and draw it:
TMultiGraph *mg = new TMultiGraph();
mg->Add(gr1);
mg->Add(gr2);
mg->Draw("ALP");
}
Figure: Graph with error bars.
Drawing a TMultiGraph
TMultiGraph drawing is similar to graph drawing and setting titles is the same. Automatic coloring and reverse axis drawing are also available.
A specific option allows to draw the graph collection in 3D.
When a legend is created using TPad::BuildLegend all
the graphs collected in a TMultiGraph
are
added in the legend.
Zooming a TMultiGraph
TMultiGraph
limits are automatically computed from the ranges of
the graphs building it. It is also possible to impose axis limits.
Fitting a TMultiGraph
When graphs are stored in a TMultiGraph
they are considered as
a single set of point by the fitting mechanism.
Automatic coloring
When several graphs are drawn on the same plot, it is possible to pick automatically the color of each graph in the current color palette.
TGraphQQ
A TGraphQQ
allows to draw quantile-quantile plots. Such plots can
be drawn for two datasets, or for one dataset and a theoretical distribution function.
TGraph2D
A TGraph2D
graph is a graphics object that is made of three
arrays X
, Y
and Z
with the same number of points each.
TGraph2DErrors
derives from TGraph2D
. It
adds 3 extra vectors to define the errors.
Creating a TGraph2D
- Use one of the
TGraph2D
constructors to create a 2D graph.
TGraph2D
(and TGraph2DErrors
) have several
constructors. For instances
from three arrays x
, y
, and z
(can be arrays of doubles, floats, or integers),
from an ASCII file, or even
without parameter (in that case use the SetPoint()
method to
fill the internal arrays).
Drawing a TGraph2D
You can draw a TGraph2D
with any
drawing option valid for 2D histogram.
In this case, an intermediate 2D histogram is filled using the Delaunay triangles technique to
interpolate the data set.
You can also use the specific TGraph2D drawing options
Example
{
auto c = new TCanvas("c","Graph2D example",0,0,700,600);
double x, y, z, P = 6.;
int np = 200;
auto dt = new TGraph2D();
auto r = new TRandom();
for (int N=0; N<np; N++) {
x = 2*P*(r->Rndm(N))-P;
y = 2*P*(r->Rndm(N))-P;
z = (sin(x)/x)*(sin(y)/y)+0.2;
dt->SetPoint(N,x,y,z);
}
dt->Draw("tri1 p0");
}
Figure: A TGraph2D with the drawing option TRI1 and P0.
Fitting a TGraph2D
Some tutorials show how to fit TGraph2D and TGraph2DErrors.